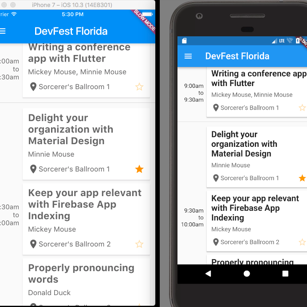
From Java to Kotlin
17 Nov 2017
android ios programming development dart flutterModeling your data with Kotlin
Modeling your data is an important part of any software project. We model our data to mimic relationships between objects and represent our database. For example, in Tasty Versions, we model our version with the **TastyVersionModel** class. package com.raywenderlich.tastyversions.models; public class TastyVersionModel { private String name; private String apiVersion; private int imageResource; public TastyVersionModel(String name, String apiVersion, int imageResource) { this.name = name; this.apiVersion = apiVersion; this.imageResource = imageResource; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getApiVersion() { return apiVersion; } public void setApiVersion(String apiVersion) { this.apiVersion = apiVersion; } public int getImageResource() { return imageResource; } public void setImageResource(int imageResource) { this.imageResource = imageResource; } } Our model allows us to have a name of type String, an API version of type String and a resource ID reference to an image that represents our version. We have a constructor that takes, as parameters, all of these values. Then we have matching getter and setter methods for each of our class fields. These 36 lines of code define what we call our Tasty Version model. That's fairly compact, but it's also a very simple class. Let's take a look at what this looks like if we were to write this class out in Kotlin. package com.raywenderlich.tastyversions.models data class TastyVersionModel(var name: String, var apiVersion: String, var imageResource: Int) That's it! With Kotlin, we can define our simple Tasty Version model by defining our primary constructor as well as our class fields with one line of code. There's something we've not seen before and that's the **data** keyword. Adding the data keyword to the class definition makes this a **data class**. This keyword tells the compiler that we want some extra functionality without having to explicitly code it. Namely, we want the following methods:- equals()
- hashCode()
- toString()
- copy()
- componentN()